stouputils.io module¶
This module provides utilities for file management.
replace_tilde: Replace the “~” by the user’s home directory
clean_path: Clean the path by replacing backslashes with forward slashes and simplifying the path
super_open: Open a file with the given mode, creating the directory if it doesn’t exist (only if writing)
super_copy: Copy a file (or a folder) from the source to the destination (always create the directory)
super_json_load: Load a JSON file from the given path
super_json_dump: Writes the provided data to a JSON file with a specified indentation depth.
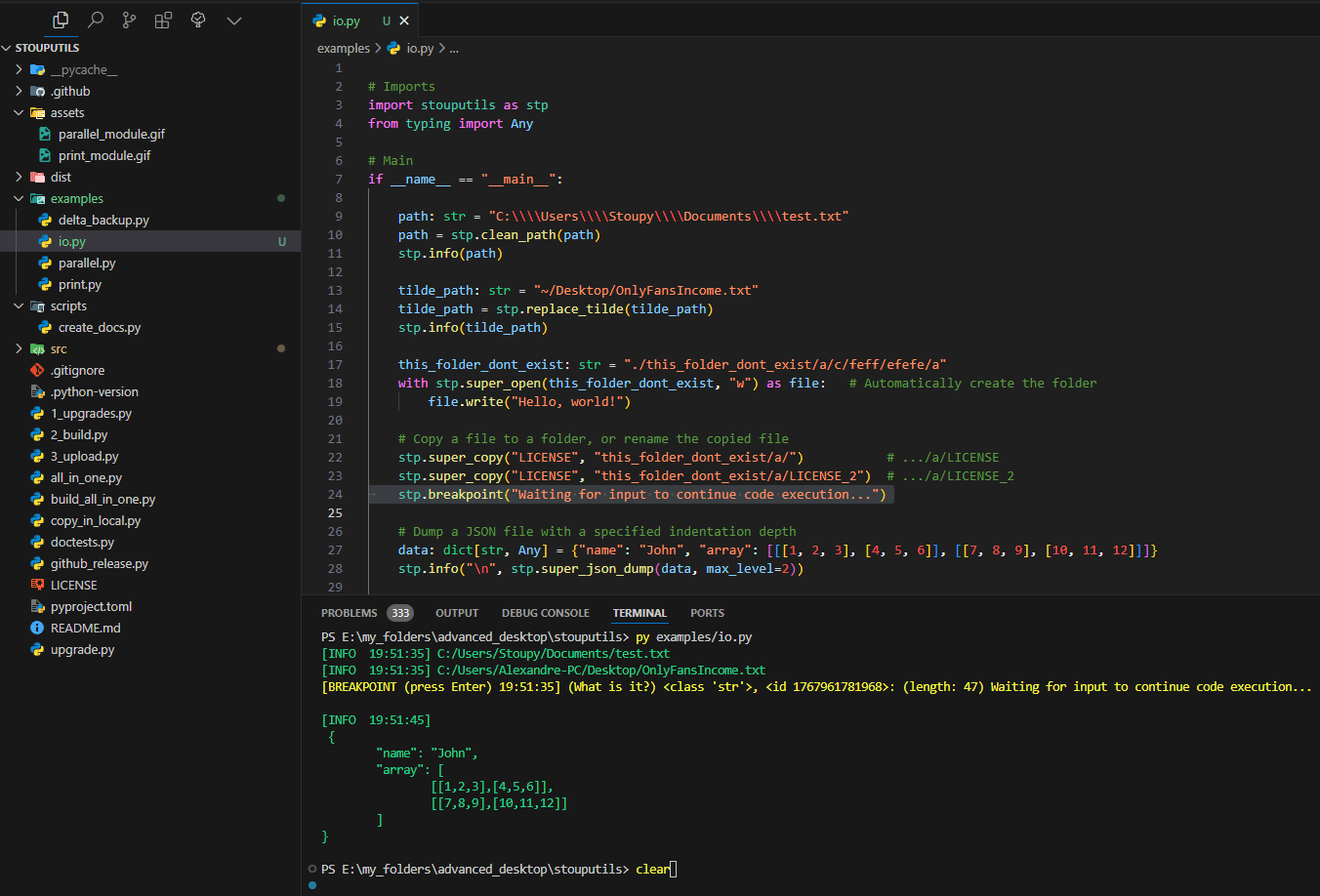
- replace_tilde(path: str) str [source]¶
Replace the “~” by the user’s home directory
- Parameters:
path (str) – The path to replace the “~” by the user’s home directory
- Returns:
The path with the “~” replaced by the user’s home directory
- Return type:
str
Examples
> replace_tilde("~/Documents/test.txt") '/home/user/Documents/test.txt'
- clean_path(file_path: str) str [source]¶
Clean the path by replacing backslashes with forward slashes and simplifying the path
- Parameters:
file_path (str) – The path to clean
- Returns:
The cleaned path
- Return type:
str
Examples
>>> clean_path("C:\\Users\\Stoupy\\Documents\\test.txt") 'C:/Users/Stoupy/Documents/test.txt'
>>> clean_path("Some Folder////") 'Some Folder/'
>>> clean_path("test/uwu/1/../../") 'test/'
>>> clean_path("some/./folder/../") 'some/'
>>> clean_path("folder1/folder2/../../folder3") 'folder3'
>>> clean_path("./test/./folder/") 'test/folder/'
>>> clean_path("C:/folder1\\folder2") 'C:/folder1/folder2'
- get_root_path(relative_path: str, go_up: int = 0) str [source]¶
Get the absolute path of the directory. Usually used to get the root path of the project using the __file__ variable.
- Parameters:
relative_path (str) – The path to get the absolute directory path from
go_up (int) – Number of parent directories to go up (default: 0)
- Returns:
The absolute path of the directory
- Return type:
str
Examples
> get_root_path(__file__) 'C:/Users/Alexandre-PC/AppData/Local/Programs/Python/Python310/lib/site-packages/stouputils' > get_root_path(__file__, 3) 'C:/Users/Alexandre-PC/AppData/Local/Programs/Python/Python310'
- super_open(file_path: str, mode: str, encoding: str = 'utf-8') IO[Any] [source]¶
Open a file with the given mode, creating the directory if it doesn’t exist (only if writing)
- Parameters:
file_path (str) – The path to the file
mode (str) – The mode to open the file with, ex: “w”, “r”, “a”, “wb”, “rb”, “ab”
encoding (str) – The encoding to use when opening the file (default: “utf-8”)
- Returns:
The file object, ready to be used
- Return type:
open
- super_copy(src: str, dst: str, create_dir: bool = True) str [source]¶
Copy a file (or a folder) from the source to the destination
- Parameters:
src (str) – The source path
dst (str) – The destination path
create_dir (bool) – Whether to create the directory if it doesn’t exist (default: True)
- Returns:
The destination path
- Return type:
str
- super_json_load(file_path: str) Any [source]¶
Load a JSON file from the given path
- Parameters:
file_path (str) – The path to the JSON file
- Returns:
The content of the JSON file
- Return type:
Any
- super_json_dump(data: Any, file: TextIOWrapper | None = None, max_level: int = 2, indent: str = '\t') str [source]¶
Writes the provided data to a JSON file with a specified indentation depth. For instance, setting max_level to 2 will limit the indentation to 2 levels.
- Parameters:
data (Any) – The data to dump (usually a dict or a list)
file (io.TextIOWrapper) – The file to dump the data to, if None, the data is returned as a string
max_level (int) – The depth of indentation to stop at (-1 for infinite)
indent (str) – The indentation character (default: ‘ ‘)
- Returns:
The content of the file in every case
- Return type:
str
>>> super_json_dump({"a": [[1,2,3]], "b": 2}, max_level = 2) '{\n\t"a": [\n\t\t[1,2,3]\n\t],\n\t"b": 2\n}\n'