stouputils.applications.automatic_docs module¶
Sphinx documentation generation utilities.
This module provides a comprehensive set of utilities for automatically generating and managing Sphinx documentation for Python projects. It handles the creation of configuration files, index pages, version management, and HTML generation.
Example of usage:
import stouputils as stp
from stouputils.applications import automatic_docs
if __name__ == "__main__":
automatic_docs.update_documentation(
root_path=stp.get_root_path(__file__, go_up=1),
project="stouputils",
author="Stoupy",
copyright="2025, Stoupy",
html_logo="https://avatars.githubusercontent.com/u/35665974",
html_favicon="https://avatars.githubusercontent.com/u/35665974",
github_user="Stoupy51",
github_repo="stouputils",
version="1.2.0",
skip_undocumented=True,
)
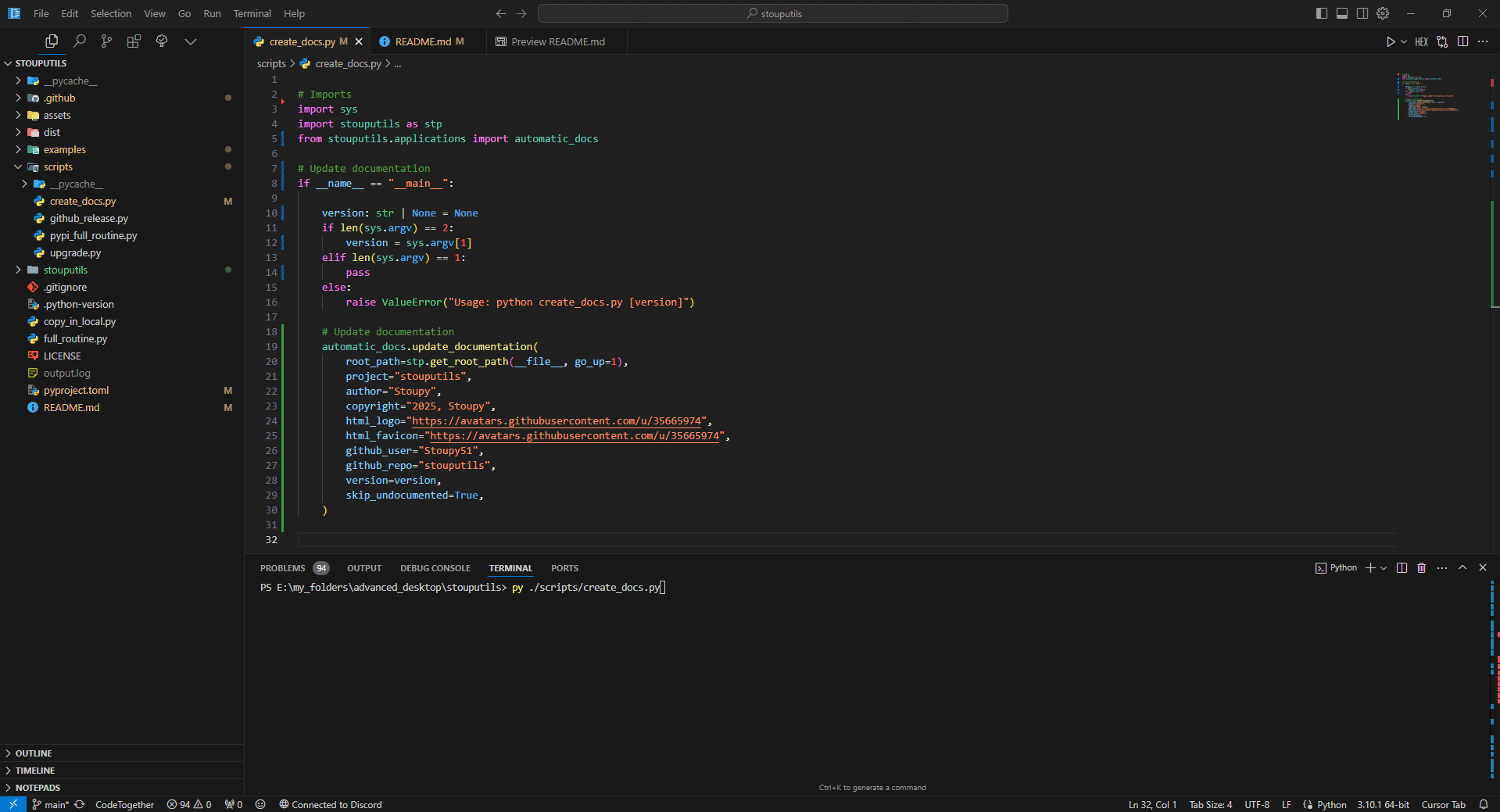
Example of GitHub Actions workflow:
name: documentation
on:
push:
tags:
- 'v*'
workflow_dispatch:
permissions:
contents: write
jobs:
docs:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v4
- uses: actions/setup-python@v5
- name: Install dependencies
run: |
pip install hatch stouputils
hatch build
- name: Build version docs
run: |
python scripts/create_docs.py ${GITHUB_REF#refs/tags/v}
- name: Deploy to GitHub Pages
uses: peaceiris/actions-gh-pages@v3
with:
publish_branch: gh-pages
github_token: ${{ secrets.GITHUB_TOKEN }}
publish_dir: docs/build/html
keep_files: true
force_orphan: false
- get_sphinx_conf_content(project: str, author: str, current_version: str, copyright: str, html_logo: str, html_favicon: str, github_user: str, github_repo: str, version_list: list[str] | None = None, skip_undocumented: bool = True) str [source]¶
Get the content of the Sphinx configuration file.
- Parameters:
project (str) – Name of the project
author (str) – Author of the project
current_version (str) – Current version
copyright (str) – Copyright information
html_logo (str) – URL to the logo
html_favicon (str) – URL to the favicon
github_user (str) – GitHub username
github_repo (str) – GitHub repository name
version_list (list[str] | None) – List of versions. Defaults to None
skip_undocumented (bool) – Whether to skip undocumented members. Defaults to True
- Returns:
Content of the Sphinx configuration file
- Return type:
str
- get_versions_from_github(github_user: str, github_repo: str) list[str] [source]¶
Get list of versions from GitHub gh-pages branch.
- Parameters:
github_user (str) – GitHub username
github_repo (str) – GitHub repository name
- Returns:
List of versions, with ‘latest’ as first element
- Return type:
list[str]
- markdown_to_rst(markdown_content: str) str [source]¶
Convert markdown content to RST format.
- Parameters:
markdown_content (str) – Markdown content
- Returns:
RST content
- Return type:
str
- generate_index_rst(readme_path: str, index_path: str, project: str, github_user: str, github_repo: str, get_versions_function: ~typing.Callable[[str, str], list[str]] = <function get_versions_from_github>) None [source]¶
Generate index.rst from README.md content.
- Parameters:
readme_path (str) – Path to the README.md file
index_path (str) – Path where index.rst should be created
project (str) – Name of the project
github_user (str) – GitHub username
github_repo (str) – GitHub repository name
get_versions_function (Callable[[str, str], list[str]]) – Function to get versions from GitHub
- generate_documentation(source_dir: str, modules_dir: str, project_dir: str, build_dir: str) None [source]¶
Generate documentation using Sphinx.
- Parameters:
source_dir (str) – Source directory
modules_dir (str) – Modules directory
project_dir (str) – Project directory
build_dir (str) – Build directory
- generate_redirect_html(filepath: str) None [source]¶
Generate HTML content for redirect page.
- Parameters:
filepath (str) – Path to the file where the HTML content should be written
- update_documentation(root_path: str, project: str, project_dir: str = '', author: str = 'Author', copyright: str = '2025, Author', html_logo: str = '', html_favicon: str = '', github_user: str = '', github_repo: str = '', version: str | None = None, skip_undocumented: bool = True, get_versions_function: ~typing.Callable[[str, str], list[str]] = <function get_versions_from_github>, generate_index_function: ~typing.Callable[[...], None] = <function generate_index_rst>, generate_docs_function: ~typing.Callable[[...], None] = <function generate_documentation>, generate_redirect_function: ~typing.Callable[[str], None] = <function generate_redirect_html>, get_conf_content_function: ~typing.Callable[[...], str] = <function get_sphinx_conf_content>) None [source]¶
Update the Sphinx documentation.
- Parameters:
root_path (str) – Root path of the project
project (str) – Name of the project
project_dir (str) – Path to the project directory (to be used with generate_docs_function)
author (str) – Author of the project
copyright (str) – Copyright information
html_logo (str) – URL to the logo
html_favicon (str) – URL to the favicon
github_user (str) – GitHub username
github_repo (str) – GitHub repository name
version (str | None) – Version to build documentation for (e.g. “1.0.0”, defaults to “latest”)
skip_undocumented (bool) – Whether to skip undocumented members. Defaults to True
get_versions_function (Callable[[str, str], list[str]]) – Function to get versions from GitHub
generate_index_function (Callable[..., None]) – Function to generate index.rst
generate_docs_function (Callable[..., None]) – Function to generate documentation
generate_redirect_function (Callable[[str], None]) – Function to create redirect file
get_conf_content_function (Callable[..., str]) – Function to get Sphinx conf.py content