stouputils.decorators module¶
This module provides decorators for various purposes:
silent(): Make a function silent (disable stdout, and stderr if specified) (alternative to stouputils.ctx.Muffle)
measure_time(): Measure the execution time of a function and print it with the given print function
handle_error(): Handle an error with different log levels
simple_cache(): Easy cache function with parameter caching method
deprecated(): Mark a function as deprecated
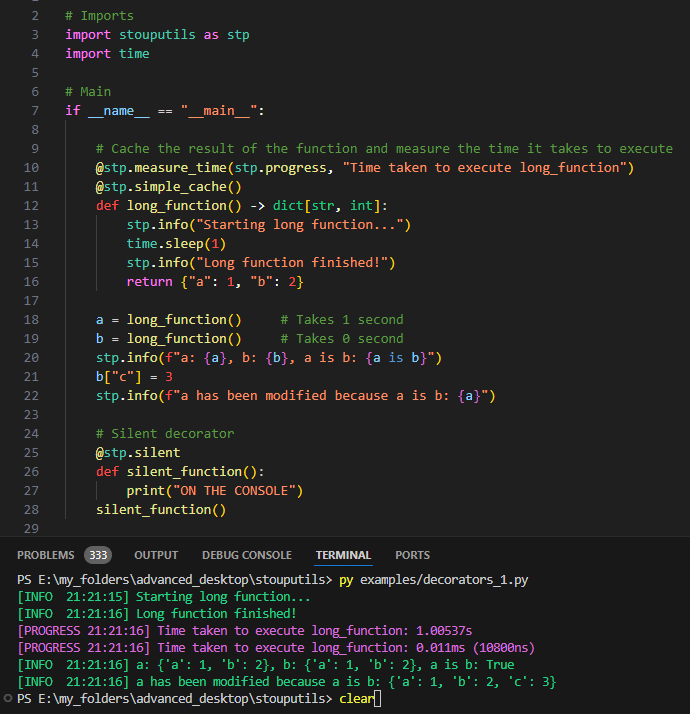

- silent(func: Callable[[...], Any], mute_stderr: bool = False) Callable[[...], Any] [source]¶
Decorator that makes a function silent (disable stdout, and stderr if specified).
Alternative to stouputils.ctx.Muffle.
- Parameters:
func (Callable[..., Any]) – Function to make silent
mute_stderr (bool) – Whether to mute stderr or not
Examples
>>> @silent ... def test(): ... print("Hello, world!") >>> test()
>>> silent(print)("Hello, world!")
- measure_time(print_func: ~typing.Callable[[...], None] = <function debug>, message: str = '', perf_counter: bool = True) Callable[[...], Any] [source]¶
Decorator that will measure the execution time of a function and print it with the given print function
- Parameters:
print_func (Callable) – Function to use to print the execution time (e.g. debug, info, warning, error, etc.)
message (str) – Message to display with the execution time (e.g. “Execution time of Something”), defaults to “Execution time of {func.__name__}”
perf_counter (bool) – Whether to use time.perf_counter_ns or time.time_ns
- Returns:
Decorator to measure the time of the function.
- Return type:
Callable
Examples
> @measure_time(info) > def test(): > pass > test() # [INFO HH:MM:SS] Execution time of test: 0.000ms (400ns)
- class LogLevels(value)[source]¶
Bases:
Enum
Log level for the errors in the decorator handle_error()
- NONE = 0¶
Do nothing
- WARNING = 1¶
Show as warning
- WARNING_TRACEBACK = 2¶
Show as warning with traceback
- ERROR_TRACEBACK = 3¶
Show as error with traceback
- RAISE_EXCEPTION = 4¶
Raise exception
- force_raise_exception: bool = False¶
If true, the error_log parameter will be set to RAISE_EXCEPTION for every next handle_error calls, useful for doctests
- handle_error(exceptions: tuple[type[BaseException], ...] | type[BaseException] = (<class 'Exception'>,), message: str = '', error_log: ~stouputils.decorators.LogLevels = LogLevels.WARNING_TRACEBACK) Callable[[...], Any] [source]¶
Decorator that handle an error with different log levels.
- Parameters:
exceptions (tuple[type[BaseException]], ...) – Exceptions to handle
message (str) – Message to display with the error. (e.g. “Error during something”)
error_log (LogLevels) – Log level for the errors LogLevels.NONE: None LogLevels.WARNING: Show as warning LogLevels.WARNING_TRACEBACK: Show as warning with traceback LogLevels.ERROR_TRACEBACK: Show as error with traceback LogLevels.RAISE_EXCEPTION: Raise exception (as if the decorator didn’t exist)
Examples
> @handle_error(error_log=LogLevels.WARNING) > def test(): > raise ValueError("Let's fail") > test() # [WARNING HH:MM:SS] Error during test: (ValueError) Let's fail
- simple_cache(method: Literal['str', 'pickle'] = 'str') Callable[[...], Callable[[...], Any]] [source]¶
Decorator that caches the result of a function based on its arguments.
The str method is often faster than the pickle method (by a little).
- Parameters:
method (Literal["str", "pickle"]) – The method to use for caching.
- Returns:
A decorator that caches the result of a function.
- Return type:
Callable[…, Callable[…, Any]]
Examples
>>> @simple_cache(method="str") ... def test(a: int, b: int) -> int: ... return a + b >>> test(1, 2) # 3 3 >>> test(1, 2) # 3 3 >>> test(3, 4) # 7 7
- deprecated(message: str = '', error_log: LogLevels = LogLevels.WARNING) Callable[[...], Any] [source]¶
Decorator that marks a function as deprecated.
- Parameters:
message (str) – Additional message to display with the deprecation warning
error_log (LogLevels) – Log level for the deprecation warning LogLevels.NONE: None LogLevels.WARNING: Show as warning LogLevels.WARNING_TRACEBACK: Show as warning with traceback LogLevels.ERROR_TRACEBACK: Show as error with traceback LogLevels.RAISE_EXCEPTION: Raise exception
- Returns:
Decorator that marks a function as deprecated
- Return type:
Callable[…, Any]
Examples
> @deprecated(message="Use 'this_function()' instead", error_log=LogLevels.WARNING) > def test(): > pass > test() # [WARNING HH:MM:SS] Function 'test()' is deprecated. Use 'this_function()' instead